Throughout my career, there have been fierce debates about style and formatting when it comes to writing code. Great holy wars were waged in support of 3-space tabs rather than 4-space. Many wounds were awarded in the struggle between dangling curly brace rather than giving the brace its very own line.
It might seem silly, and certainly those issues were, but in the end, the formatting that we provide to our code can make the difference between understanding the operation with a casual inspection vs. understanding only after careful and lengthy consideration.
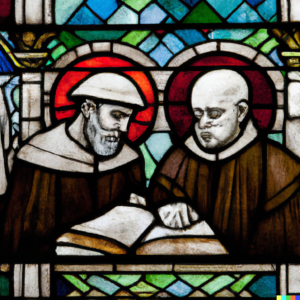
With languages that afford the programmer with great latitude around formatting and style, there are often vast differences in how the code looks, and how easily it might be maintained. Perl for instance, was so free-range that it was often called a “write-only” language. In fact there was a conspicuous competition each year that awarded the top prize for the most unreadable code. (The Obfuscated Perl contest.)
When it comes to DataWeave, although there is no competition for it, there are practitioners who act as if they are warmly invited to obfuscate the meaning of their expressions. You can see it in this example:
Take a look at this code example and see if the meaning is apparent, or even available.
var flights = payload.flights.*flight fun allRevenue(f:Array) = f reduce (e,a=0) ->
a + (e.price * e."available-seats") var score = {delta: {seats:0,revenue:0},
united: {seats:0,revenue:0},american: {seats:0,revenue:0}
} ---
flights reduce (fl,acc=score) -> fl match {case delta if (fl."airline-name"
== "delta") -> acc update { case del at .delta -> { seats: acc.delta.seats
+ fl."available-seats",revenue: acc.delta.revenue + fl."available-seats" *
fl.price }} case american if (fl."airline-name" == "american" ) -> acc
update {case amer at .american -> {seats: acc.american.seats + fl."available-seats",
revenue: acc.american.revenue + fl."available-seats" * fl.price } } case united
if (fl."airline-name" == "united") -> acc update { case united at .united ->
{seats: acc.united.seats + fl."available-seats", revenue: acc.united.revenue
+ fl."available-seats" * fl.price }} else -> acc}
Yeah, if you can indeed read that, you are an accomplished master of the craft.
Okay, perhaps that overstates the case, but certainly this code is not positioned for someone to debug it or add features. Nope, this is pretty much the same as black box code.
So how should it look if we want it to be understood later in time, or by another developer? There is no one right answer, but consider this one:
var flights = payload.flights.*flight
fun allRevenue(f:Array) =
f reduce (e,a=0) ->
a + (e.price * e."available-seats")
var score = {
delta: {seats:0,revenue:0},
united: {seats:0,revenue:0},
american: {seats:0,revenue:0}
}
---
//allRevenue(flights)
flights reduce (fl,acc=score) ->
fl match {
case delta if (fl."airline-name" == "delta") ->
acc update {
case del at .delta -> {
seats: acc.delta.seats + fl."available-seats",
revenue: acc.delta.revenue + fl."available-seats" * fl.price
}
}
case american if (fl."airline-name" == "american") ->
acc update {
case amer at .american -> {
seats: acc.american.seats + fl."available-seats",
revenue: acc.american.revenue + fl."available-seats" * fl.price
}
}
case united if (fl."airline-name" == "united") ->
acc update {
case united at .united -> {
seats: acc.united.seats + fl."available-seats",
revenue: acc.united.revenue + fl."available-seats" * fl.price
}
}
else -> acc
}
Even without seeing the sample data that represents “payload” it is possible to derive a lot, simply by inspecting this code.
var flights = payload.flights.*flight
We see the use of the multi-value selector and that will give us an Array.
The definition of the “score” variable stands out and can easily be traced to its use as the accumulator in the reduce()
call a few lines later.
var score = {
delta: {seats:0,revenue:0},
united: {seats:0,revenue:0},
american: {seats:0,revenue:0}
}
The conditions for the case constructs inside the match()
call are easy to identify and understand.
case delta if (fl."airline-name" == "delta") ->
acc update {
In fact, we can see by examining the entire expression, that we might build the match()
call with one case and a default. Look for the repetition, and speculate about how it can be collapsed.
You do not have to agree with my specific presentation style here, but I hope you will agree that readability improves the value of the code.
The main point is that every block of code communicates on two axes. It contains instructions to be executed by a computing device, and it expresses its intention to the person who will need to maintain it.
Experience shows that for my code, that person will probably be me.
So when you write code, maybe — be nice to yourself.